Overview
Dynamic Import
Our dynamic import feature allows you to start the nuvo importer at any step, at any event, and with your preferred data format. Use the flexibility of this option to cover all your use cases:
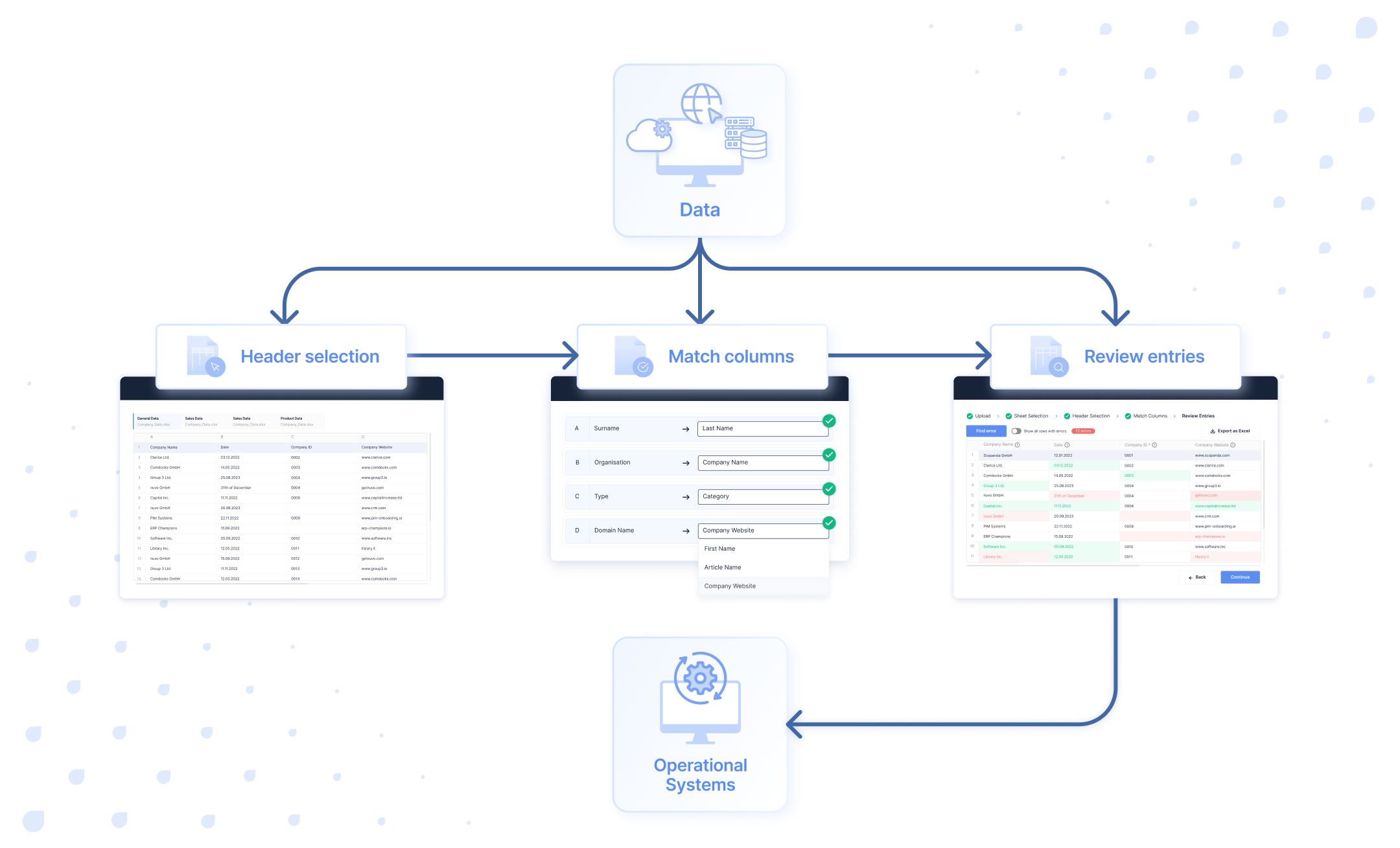
Description | This feature provides the flexibility to initiate the data import process at different stages, allowing you to customize the importer's behavior according to your requirements. Instead of starting the importer solely during the upload step, you can now choose to begin it during the header, mapping, or review steps. Additionally, you have the ability to define when the importer should open, expanding beyond the initial import performed by the user. By leveraging this feature, you can trigger the importing process based on various events, such as a specific time event, a user interaction, or the reception of data from an external source. This means you are no longer limited to manually importing data, and you can utilize data obtained from your API or any third-party service. Whether it's fetched data or information already present in the browser, you can leverage it to initiate the importer. These capabilities empower you to construct your own workflow, providing unparalleled flexibility in how you handle the data import process. |
Overall, the Dynamic Import feature provides a lot of flexibility and customization options for the nuvo Importer SDK. By allowing you to start the import process dynamically and choose your own workflow, you can save time and improve your productivity. | |
Run Event | Based on your configuration, this feature allows you to begin the import process from either the "Upload" step, "Sheet Selection" step, “Header Selection” step, “Match Columns” step, or “Review Entries” step. |
Parameter | nuvoSession.parse(): This function enables you to parse one or multiple input files. The output is an object with two keys: accepted and rejected . accepted is an array of file objects from successfully parsed files, with each object containing a fileName and a sheets key. sheets holds an array of sheet objects, each with a sheetName and data key. rejected contains an array of file objects that were rejected. Files can be rejected because their file type is not supported, they are not parsable, their content is invalid, or parsing for a certain file type is not part of your subscription. Before passing this output to nuvoSession.upload() , ensure it has been modified to match the correct input structure. Note that the input format may vary depending on the step you start from, such as "Sheet Selection", "Header Selection", "Match Columns", or "Review Entries".
|
nuvoSession.upload(): This function enables you to upload data either in one go or in multiple parts.
| |
nuvoSession.start(): The function enables you to start the import process from their preferred step.
| |
Implementation Example | In the given example, the data is fetched from the API and the import process is started dynamically. |
- React
- Angular
- Vue
- JavaScript
import { NuvoImporter, nuvoSession } from "nuvo-react";
import React, { useEffect } from "react";
function App() {
const files = [];
useEffect(() => {
const processFiles = async () => {
try {
const fileData = await nuvoSession.parse(files, "product_data");
nuvoSession.upload(
{
step: "sheetSelection",
data: fileData.accepted,
headerIndex: undefined,
},
"product_data",
);
nuvoSession.start("product_data");
} catch (error) {
console.error("Error processing files:", error);
}
};
processFiles();
}, []);
return (
<div className="App">
<NuvoImporter
licenseKey="Your License Key"
settings={{
developerMode: true,
identifier: "product_data",
modal: true,
columns: [],
}}
onResults={(result, errors, complete, logs) => {
complete();
}}
/>
</div>
);
}
export default App;
- Add the NuvoImporter module to the file
app.module.ts
.
import { NuvoImporterModule } from "nuvo-angular";
@NgModule({
declarations: [AppComponent],
imports: [NuvoImporterModule, BrowserModule, AppRoutingModule],
providers: [],
bootstrap: [AppComponent],
})
export class AppModule {}
- Add the nuvo component inside the HTML file
app.component.html
.
<nuvo-importer [licenseKey]="licenseKey" [settings]="settings" />
- Define the property inside the component file
app.component.ts
.
import { RejectSubmitResult, ResultValues, nuvoSession } from 'nuvo-angular';
export class AppComponent implements OnInit {
settings!: SettingsAPI;
licenseKey!: string;
files = [];
openSession = async () => {
try {
const fileData = await nuvoSession.parse(files, 'product_data');
nuvoSession.upload({
step: 'sheetSelection',
data: fileData.accepted,
headerIndex: 1,
}, 'product_data');
nuvoSession.start('product_data');
} catch (error) {
console.error("Error processing files:", error);
}
};
ngOnInit(): void {
this.licenseKey = "Your license key";
this.settings = {
identifier: "product_data",
developerMode: true,
modal: true,
columns: [],
};
}
onResults = (
results: ResultValues,
identifier: string,
complete: (rejection?: RejectSubmitResult) => void
) => {
complete();
};
}
We're phasing out support for Vue 2. If you're still using Vue 2, you can use "nuvo-vuejs" v2.9 or lower.
- Add the nuvo component inside the
<template>
tag in the fileApp.vue
.
<template>
<div>
<button @click="openSession">Start session</button>
<nuvo-importer
:license-key="licenseKey"
:on-results="onResults"
:settings="settings"
/>
</div>
</template>
- Define the property inside the
<script>
tag in the component file.
import { NuvoImporter, nuvoSession } from "nuvo-vuejs";
export default {
name: "App",
components: {
NuvoImporter,
},
setup() {
const settings = {
developerMode: true,
identifier: "product_data",
modal: true,
columns: [],
};
return { settings };
},
data() {
return {
licenseKey: "Your license key",
};
},
methods: {
openSession = async () => {
try {
const fileData = await nuvoSession.parse(files, "product_data");
nuvoSession.upload(
{
step: "sheetSelection",
data: fileData.accepted,
headerIndex: 1,
},
"product_data"
);
nuvoSession.start("product_data");
} catch (error) {
console.error("Error processing files:", error);
}
};
onResults: async (result, errors, complete, logs) => {
complete();
},
},
};
Our vanilla JS syntax has changed since v2.9. If you use v2.8 or lower, please migrate to the latest version by following our migration guide.
<div class="nuvo-container" />
<button id="start-session">Import data</button>
<script type="module">
import { launchNuvoImporter, nuvoSession } from "nuvo-vanilla-js";
launchNuvoImporter(".nuvo-container", {
licenseKey: "Your License Key",
settings: {
developerMode: true,
identifier: "product_data",
modal: true,
columns: [],
},
onResults: (result, errors, complete, logs) => {
complete();
},
});
const button = document.getElementById("start-session");
button.addEventListener("click", async () => {
try {
const fileData = await nuvoSession.parse(files, "product_data");
nuvoSession.upload(
{
step: "sheetSelection",
data: fileData.accepted,
headerIndex: 1,
},
"product_data",
);
nuvoSession.start("product_data");
} catch (error) {
console.error("Error processing files:", error);
}
});
</script>
Start from upload step
Description | By incorporating the parameters described below, the dynamic import feature enables you to initiate the import process directly from the upload step seamlessly. |
Parameter | nuvoSession.upload(): Not required |
nuvoSession.start(): Calling this function will open the importer within the "Upload" step. |
It is only possible to open the importer from the “Upload" step if modal is set to true
inside importer settings.
Start from sheet selection step
Description | By incorporating the parameters described below, the dynamic import feature enables you to initiate the import process directly from the sheet selection step seamlessly. |
Parameter | nuvoSession.upload() : With this function, you can enable your users to upload data, and you can specify the exact step at which the importer should be initiated. |
| |
nuvoSession.start(): Calling this function will open the importer within the "Sheet Selection" step. | |
Implementation Example | The following example demonstrates the expected data format for both scenarios. |
If you want to enable your users to select multiple sheets/files at once, you need to set multipleFileUpload
to true
in the settings. Please note that this feature can only be used if it is included in your plan. If you are interested in testing or activating it, please contact your salesperson or send an email to [email protected].
- JSON Input
- 2D array Input
nuvoSession.upload(
{
step: "sheetSelection",
data: [
{
fileName: "product_data.xlsx",
sheets: [
{
sheetName: "Product Data",
data: [
{ product_id: "1", product_name: "StellarGlow Pro 2000" },
{ product_id: "2", product_name: "QuantumTune Elite X9" },
],
},
{
sheetName: "Address Data",
data: [{ street: "1234 Elm Street", city: "Meadowville", country: "Echovia" }],
},
],
},
{
fileName: "customer_data.csv",
sheets: [
{
sheetName: "Customers",
data: [{ first_name: "Harper", last_name: "Mitchell" }],
},
],
},
],
},
"Your importer's identifier",
);
nuvoSession.upload(
{
step: "sheetSelection",
data: [
{
fileName: "product_data.xlsx",
sheets: [
{
sheetName: "Product Data",
data: [
["Product id", "Product name"],
["1", "StellarGlow Pro 2000"],
["2", "QuantumTune Elite X9"],
],
},
{
sheetName: "Address Data",
data: [
["Street", "City", "Country"],
["1234 Elm Street", "Meadowville", "Echovia"],
],
},
],
},
{
fileName: "customer_data.csv",
sheets: [
{
sheetName: "Customers",
data: [
["First name", "Last name"],
["Harper", "Mitchell"],
],
},
],
},
],
},
"Your importer's identifier",
);
Start from header step
Description | By incorporating the parameters described below, the dynamic import feature enables you to initiate the import process directly from the header step seamlessly. |
Parameter | nuvoSession.upload(): With this function, you can enable your users to upload data, and you can specify the exact step at which the importer should be initiated.
|
nuvoSession.start(): Calling this function will open the importer within the "Header Selection" step. | |
Implementation Example | The following example demonstrates the expected data format for both scenarios. |
- JSON Input
- 2D array Input
nuvoSession.upload(
{
step: "header",
data: [
{ id: 1, country: "Germany" },
{ id: 2, country: "China" },
],
headerIndex: undefined,
},
"Your importer's identifier",
);
nuvoSession.upload(
{
step: "header",
data: [
["Author:", "Harper"],
["id", "country"],
[1, "germany"],
[2, "china"],
],
headerIndex: 2,
},
"Your importer's identifier",
);
Start from mapping step
Description | By incorporating the parameters described below, the dynamic import feature enables you to initiate the import process directly from the mapping step seamlessly. |
Parameter | nuvoSession.upload(): With this function, you can enable your users to upload data, and you can specify the exact step at which the importer should be initiated.
|
nuvoSession.start(): Calling this function will open the importer within the "Match Columns" step". | |
Implementation Example | The following example demonstrates the expected data format for both scenarios. |
- JSON Input
- 2D array Input
nuvoSession.upload(
{
step: "mapping",
data: [
{ id: 1, country: "Germany" },
{ id: 2, country: "China" },
],
headerIndex: undefined,
},
"Your importer's identifier",
);
nuvoSession.upload(
{
step: "mapping",
data: [
[1, "germany"],
["id", "country"],
[2, "china"],
],
headerIndex: 2,
},
"Your importer's identifier",
);
Start from review step
Description | By incorporating the parameters described below, the dynamic import feature enables you to initiate the import process directly from the review step seamlessly. |
Parameter | nuvoSession.upload(): With this function, you can enable your users to upload data, and you can specify the exact step at which the importer should be initiated.
|
nuvoSession.start(): Calling this function will open the importer within the "Review Entries" step. | |
Implementation Example | The following example demonstrates the expected data format for both scenarios. |
It is crucial to ensure that the keys in the JSON align with the keys in your target data model.
- JSON
- JSON (with errors)
nuvoSession.upload(
{
step: "review",
data: [
{ id: 1, country: "Germany" },
{ id: 2, country: "China" },
],
headerIndex: undefined,
},
"Your importer's identifier",
);
nuvoSession.upload(
{
step: "review",
data: [
{
id: {
value: 1,
info: [{ message: "Id exists in the database", level: "error" }],
},
country: {
value: "Germany",
},
},
{
id: {
value: 2,
},
country: {
value: "China",
info: [{ message: "Country does not belong to Europe", level: "info" }],
},
},
],
headerIndex: undefined,
},
"Your importer's identifier",
);
Managing multiple nuvo importer instances
In certain scenarios, you might need to manage multiple instances of nuvo importers within your application concurrently. This can be particularly beneficial when offering users the option to import data from various sources, each requiring a different output format. For instance, you could allow customers to choose whether to import product data or customer data.
Step 1: Embed the nuvo importers
To start utilizing the nuvo importers, you first need to embed them within your application. Each nuvo importer instance is defined by its unique identifier. Additionally, each instance can have its own settings and columns array, which dictates the structure of the output data.
<NuvoImporter
licenseKey="Your License Key"
settings={{
developerMode: true,
identifier: "product_data",
columns: [],
}}
/>
<NuvoImporter
licenseKey="Your License Key"
settings={{
developerMode: true,
identifier: "customer_data",
columns: [],
}}
/>
Step 2: Upload data to the respective importer
The next step involves uploading data to the specific nuvo importer instances. Each instance is identified by its unique identifier, and the uploaded data will be associated with that identifier.
// Upload data to the product instance
nuvoSession.upload(
{
step: "review",
data: [
{ product_id: "1", product_name: "StellarGlow Pro 2000" },
{ product_id: "2", product_name: "QuantumTune Elite X9" },
],
},
"product_data",
);
// Upload data to the customer instance
nuvoSession.upload(
{
step: "review",
data: [
{ customer_id: "1", customer_name: "James" },
{ customer_id: "2", customer_name: "Valentin" },
],
},
"customer_data",
);
Step 3: Start the importers
Finally, you can trigger the import process by starting the respective nuvo importer instances. The identifier
you provide to the nuvoSession.start()
method corresponds to the unique identifier of the desired nuvo importer instance. Upon triggering, the nuvo importer associated with the specified identifier will open from the defined stage, utilizing the data that was previously uploaded with the same identifier.
For instance:
nuvoSession.start("product_data"); // Opens the "product_data" importer
nuvoSession.start("customer_data"); // Opens the "customer_data" importer
By following these steps, you can seamlessly manage and utilize multiple nuvo importer instances within your application, catering to various data import needs.